This header defines the Registry messaging protocol. More...
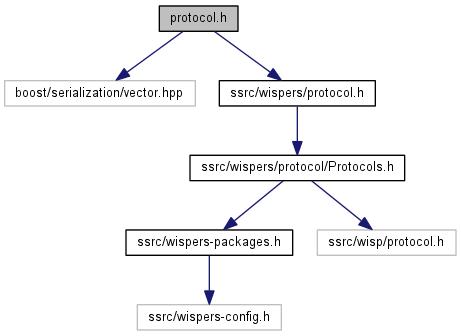
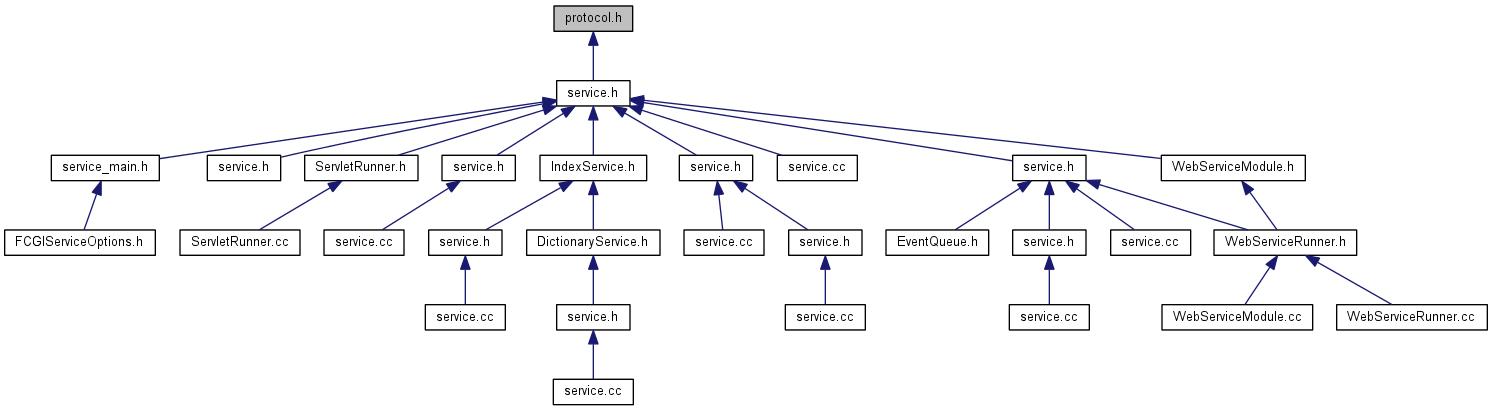
Go to the source code of this file.
Classes | |
struct | RegistryEntry |
Defines | |
#define | WSPR_MESSAGE_REGISTRATION(message_id) |
Typedefs | |
typedef boost::multi_index_container < RegistryEntry, boost::multi_index::indexed_by < boost::multi_index::hashed_non_unique < boost::multi_index::member < RegistryEntry, string,&RegistryEntry::service_name > >, boost::multi_index::hashed_non_unique < boost::multi_index::member < RegistryEntry, string,&RegistryEntry::service_type > >, boost::multi_index::hashed_unique < boost::multi_index::composite_key < RegistryEntry, boost::multi_index::member < RegistryEntry, string,&RegistryEntry::service_name > , boost::multi_index::member < RegistryEntry, string,&RegistryEntry::service_type > > > > > | service_map |
typedef service_map::nth_index < ByName >::type | index_by_name |
typedef service_map::nth_index < ByType >::type | index_by_type |
typedef service_map::nth_index < ByComposite >::type | index_by_composite |
typedef std::vector< string > | query_list |
typedef std::vector< string > | service_type_list |
typedef protocol::MessageRegistry < QueryAll > | MessageQueryAll |
typedef BinaryPackingTraits | RegistryPackingTraits |
typedef NS_SSRC_WISP_PROTOCOL::Caller < RegistryPackingTraits > | RegistryCaller |
Enumerations | |
enum | RegistryMessageType { Register, Reregister, Unregister, QueryResult, QueryByName, QueryByType, QueryAll } |
enum | IndexScheme { ByName, ByType, ByComposite } |
Functions | |
__BEGIN_NS_SSRC_WSPR_PROTOCOL | WSPR_DEFINE_PROTOCOL (Registry, registry) |
string | service_group_registry () |
Returns a string identifying the group to which all Registry instances belong. | |
string | event_group_register () |
WISP_PROTOCOL_MESSAGE (Register, protocol::MessageRegistry,((string, service_name))((service_type_list, service_types))) | |
WISP_PROTOCOL_MESSAGE (Reregister, protocol::MessageRegistry,((string, service_name))((service_type_list, service_types))) | |
WISP_PROTOCOL_MESSAGE (Unregister, protocol::MessageRegistry,((string, service_name))((service_type_list, service_types))) | |
WISP_PROTOCOL_MESSAGE (QueryResult, protocol::MessageRegistry,((service_map, result))) | |
WISP_PROTOCOL_MESSAGE (QueryByName, protocol::MessageRegistry,((query_list, keys))) | |
WISP_PROTOCOL_MESSAGE (QueryByType, protocol::MessageRegistry,((query_list, keys))) | |
WISP_ONE_WAY_CALL (RegistryCaller, Register) | |
WISP_ONE_WAY_CALL (RegistryCaller, Reregister) | |
WISP_ONE_WAY_CALL (RegistryCaller, Unregister) | |
WISP_ONE_WAY_CALL (RegistryCaller, QueryResult) | |
WISP_TWO_WAY_CALL (RegistryCaller, QueryByName, QueryResult) | |
WISP_TWO_WAY_CALL (RegistryCaller, QueryByType, QueryResult) | |
WISP_TWO_WAY_CALL (RegistryCaller, QueryAll, QueryResult) | |
template<typename caller_type > | |
string | get_service_by_type (caller_type &caller, string service_type) |
Synchronously looks up a service by its service type. |
Detailed Description
This header defines the Registry messaging protocol.
Definition in file registry/protocol.h.
Define Documentation
#define WSPR_MESSAGE_REGISTRATION | ( | message_id | ) |
WISP_PROTOCOL_MESSAGE(message_id, protocol::MessageRegistry, \ ((string, service_name)) \ ((service_type_list, service_types)))
Definition at line 111 of file registry/protocol.h.
Typedef Documentation
typedef service_map::nth_index<ByComposite>::type index_by_composite |
Definition at line 89 of file registry/protocol.h.
typedef service_map::nth_index<ByName>::type index_by_name |
Definition at line 87 of file registry/protocol.h.
typedef service_map::nth_index<ByType>::type index_by_type |
Definition at line 88 of file registry/protocol.h.
typedef protocol::MessageRegistry<QueryAll> MessageQueryAll |
Definition at line 109 of file registry/protocol.h.
typedef std::vector<string> query_list |
Definition at line 106 of file registry/protocol.h.
typedef NS_SSRC_WISP_PROTOCOL::Caller<RegistryPackingTraits> RegistryCaller |
Definition at line 130 of file registry/protocol.h.
typedef BinaryPackingTraits RegistryPackingTraits |
Definition at line 129 of file registry/protocol.h.
typedef boost::multi_index_container< RegistryEntry, boost::multi_index::indexed_by< boost::multi_index::hashed_non_unique< boost::multi_index::member<RegistryEntry, string, &RegistryEntry::service_name> >, boost::multi_index::hashed_non_unique< boost::multi_index::member<RegistryEntry, string, &RegistryEntry::service_type> >, boost::multi_index::hashed_unique< boost::multi_index::composite_key< RegistryEntry, boost::multi_index::member<RegistryEntry, string, &RegistryEntry::service_name>, boost::multi_index::member<RegistryEntry, string, &RegistryEntry::service_type> > > > > service_map |
Definition at line 84 of file registry/protocol.h.
typedef std::vector<string> service_type_list |
Definition at line 107 of file registry/protocol.h.
Enumeration Type Documentation
enum IndexScheme |
Definition at line 64 of file registry/protocol.h.
enum RegistryMessageType |
Definition at line 59 of file registry/protocol.h.
Function Documentation
string event_group_register | ( | ) | [inline] |
Definition at line 102 of file registry/protocol.h.
References WSPR_EVENT_GROUP.
string get_service_by_type | ( | caller_type & | caller, |
string | service_type | ||
) |
Synchronously looks up a service by its service type.
- Parameters:
-
caller The Caller to use to perform the lookup. service_type A string identifying the service type to look up.
- Returns:
- The private group name of the first service found matching the specified type. If no service is found, returns an empty string.
Definition at line 152 of file registry/protocol.h.
References service_group_registry(), and string.
string service_group_registry | ( | ) | [inline] |
Returns a string identifying the group to which all Registry instances belong.
This is the group where clients should direct their queries.
- Returns:
- A string identifying the group to which all Registry instances belong.
Definition at line 98 of file registry/protocol.h.
Referenced by get_service_by_type(), and ServiceProtocolProcessor::transition().
WISP_PROTOCOL_MESSAGE | ( | Register | , |
protocol::MessageRegistry | , | ||
((string, service_name))((service_type_list, service_types)) | |||
) |
WISP_PROTOCOL_MESSAGE | ( | Reregister | , |
protocol::MessageRegistry | , | ||
((string, service_name))((service_type_list, service_types)) | |||
) |
WISP_PROTOCOL_MESSAGE | ( | Unregister | , |
protocol::MessageRegistry | , | ||
((string, service_name))((service_type_list, service_types)) | |||
) |
WISP_PROTOCOL_MESSAGE | ( | QueryResult | , |
protocol::MessageRegistry | , | ||
((service_map, result)) | |||
) |
WISP_PROTOCOL_MESSAGE | ( | QueryByName | , |
protocol::MessageRegistry | , | ||
((query_list, keys)) | |||
) |
WISP_PROTOCOL_MESSAGE | ( | QueryByType | , |
protocol::MessageRegistry | , | ||
((query_list, keys)) | |||
) |
__BEGIN_NS_SSRC_WSPR_PROTOCOL WSPR_DEFINE_PROTOCOL | ( | Registry | , |
registry | |||
) |