#include <GroupSessionDatabase.h>
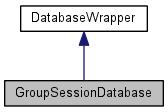
Public Types | |
typedef RowOperations < GroupSession > | GroupSessionOperations |
typedef RowOperations< Member > | MemberOperations |
typedef RowOperations < Reservation > | ReservationOperations |
Public Member Functions | |
GroupSessionDatabase (const string &db_file, const Properties &properties) SSRC_DECL_THROW(DatabaseException) | |
virtual | ~GroupSessionDatabase () |
unsigned int | count_sessions () const SSRC_DECL_THROW(DatabaseException) |
unsigned int | count_reservations () const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_participant (const functor &apply, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_participants (container_type &results, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_participant_session (const functor &apply, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_participant_sessions (container_type &results, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_observer_session (const functor &apply, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_observer_sessions (container_type &results, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_member_for_uid (const functor &apply, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_members_for_uid (container_type &results, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_member_for_participant (const functor &apply, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_members_for_participant (container_type &results, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_observer (const functor &apply, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_observers (container_type &results, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
unsigned int | remove_observer_by_uid (const uid_type observer) |
template<typename iterator_type > | |
unsigned int | remove_observers_by_uid (const iterator_type &begin, const iterator_type &end) |
template<typename functor > | |
unsigned int | for_each_member_uid (const functor &apply, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_member_uids (container_type &results, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_member_uid_discard (const functor &apply, const gsid_type gsid, const uid_type discard) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_member_uids_discard (container_type &results, const gsid_type gsid, const uid_type discard) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_expired_gsid (const functor &apply, const sec_type expires) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_expired_gsids (container_type &results, const sec_type expires) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_session_by_type (const functor &apply, const string &type) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_sessions_by_type (container_type &results, const string &type) const SSRC_DECL_THROW(DatabaseException) |
unsigned int | remove_expired_sessions (const sec_type expires) SSRC_DECL_THROW(DatabaseException) |
unsigned int | remove_reservation (const gsid_type gsid, const uid_type creator) SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_reservation_participant (const functor &apply, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_reservation_participants (container_type &results, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
unsigned int | remove_expired_reservations (const sec_type expires) SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_participant_reservation (const functor &apply, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | get_participant_reservations (container_type &results, const uid_type uid) const SSRC_DECL_THROW(DatabaseException) |
template<typename functor > | |
unsigned int | for_each_session (const functor &apply, const db_limit_type limit=-1, const db_offset_type offset=0) const SSRC_DECL_THROW(DatabaseException) |
template<typename container_type > | |
unsigned int | find_sessions (container_type &results, const db_limit_type limit=-1, const db_offset_type offset=0) const SSRC_DECL_THROW(DatabaseException) |
bool | is_member (const uid_type member, const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
unsigned int | count_observers (const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
unsigned int | activate_reservation_participants (const gsid_type gsid) const SSRC_DECL_THROW(DatabaseException) |
void | begin_transaction () SSRC_DECL_THROW(DatabaseException) |
void | rollback_transaction () SSRC_DECL_THROW(DatabaseException) |
void | end_transaction () SSRC_DECL_THROW(DatabaseException) |
Static Public Member Functions | |
static unsigned int | remove_member_by_uid (PreparedStatement &query, const uid_type member) SSRC_DECL_THROW(DatabaseException) |
template<typename iterator_type > | |
static unsigned int | remove_members_by_uid (PreparedStatement &query, const iterator_type &begin, const iterator_type &end) SSRC_DECL_THROW(DatabaseException) |
Public Attributes | |
GroupSessionOperations | group_session_ops |
MemberOperations | participant_ops |
MemberOperations | observer_ops |
ReservationOperations | reservation_ops |
MemberOperations | reservation_participant_ops |
Protected Attributes | |
Database | _database |
Friends | |
class | DatabaseTransaction |
Detailed Description
Definition at line 40 of file GroupSessionDatabase.h.
Member Typedef Documentation
Definition at line 66 of file GroupSessionDatabase.h.
typedef RowOperations<Member> GroupSessionDatabase::MemberOperations |
Definition at line 67 of file GroupSessionDatabase.h.
typedef RowOperations<Reservation> GroupSessionDatabase::ReservationOperations |
Definition at line 68 of file GroupSessionDatabase.h.
Constructor & Destructor Documentation
GroupSessionDatabase::GroupSessionDatabase | ( | const string & | db_file, |
const Properties & | properties | ||
) | [inline] |
Definition at line 76 of file GroupSessionDatabase.h.
References GSDB_INIT_QUERY.
virtual GroupSessionDatabase::~GroupSessionDatabase | ( | ) | [inline, virtual] |
Definition at line 123 of file GroupSessionDatabase.h.
Member Function Documentation
unsigned int GroupSessionDatabase::activate_reservation_participants | ( | const gsid_type | gsid | ) | const [inline] |
Definition at line 442 of file GroupSessionDatabase.h.
References gsid().
void DatabaseWrapper::begin_transaction | ( | ) | [inline, inherited] |
Definition at line 49 of file DatabaseTransaction.h.
References DatabaseWrapper::_database.
unsigned int GroupSessionDatabase::count_observers | ( | const gsid_type | gsid | ) | const [inline] |
Definition at line 433 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::count_reservations | ( | ) | const [inline] |
Definition at line 156 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::count_sessions | ( | ) | const [inline] |
Definition at line 149 of file GroupSessionDatabase.h.
void DatabaseWrapper::end_transaction | ( | ) | [inline, inherited] |
Definition at line 57 of file DatabaseTransaction.h.
References DatabaseWrapper::_database.
unsigned int GroupSessionDatabase::find_expired_gsids | ( | container_type & | results, |
const sec_type | expires | ||
) | const [inline] |
Definition at line 324 of file GroupSessionDatabase.h.
References expires().
unsigned int GroupSessionDatabase::find_members_for_participant | ( | container_type & | results, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 242 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::find_members_for_uid | ( | container_type & | results, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 227 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::find_observers | ( | container_type & | results, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 259 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::find_participants | ( | container_type & | results, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 173 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::find_sessions | ( | container_type & | results, |
const db_limit_type | limit = -1 , |
||
const db_offset_type | offset = 0 |
||
) | const [inline] |
Definition at line 416 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::for_each_expired_gsid | ( | const functor & | apply, |
const sec_type | expires | ||
) | const [inline] |
Definition at line 316 of file GroupSessionDatabase.h.
References expires().
unsigned int GroupSessionDatabase::for_each_member_for_participant | ( | const functor & | apply, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 234 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::for_each_member_for_uid | ( | const functor & | apply, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 219 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::for_each_member_uid | ( | const functor & | apply, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 278 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::for_each_member_uid_discard | ( | const functor & | apply, |
const gsid_type | gsid, | ||
const uid_type | discard | ||
) | const [inline] |
Definition at line 295 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::for_each_observer | ( | const functor & | apply, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 251 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::for_each_observer_session | ( | const functor & | apply, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 200 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::for_each_participant | ( | const functor & | apply, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 165 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::for_each_participant_reservation | ( | const functor & | apply, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 389 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::for_each_participant_session | ( | const functor & | apply, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 182 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::for_each_reservation_participant | ( | const functor & | apply, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 364 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::for_each_session | ( | const functor & | apply, |
const db_limit_type | limit = -1 , |
||
const db_offset_type | offset = 0 |
||
) | const [inline] |
Definition at line 407 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::for_each_session_by_type | ( | const functor & | apply, |
const string & | type | ||
) | const [inline] |
Definition at line 333 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::get_member_uids | ( | container_type & | results, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 286 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::get_member_uids_discard | ( | container_type & | results, |
const gsid_type | gsid, | ||
const uid_type | discard | ||
) | const [inline] |
Definition at line 305 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::get_observer_sessions | ( | container_type & | results, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 209 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::get_participant_reservations | ( | container_type & | results, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 398 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::get_participant_sessions | ( | container_type & | results, |
const uid_type | uid | ||
) | const [inline] |
Definition at line 191 of file GroupSessionDatabase.h.
References uid().
unsigned int GroupSessionDatabase::get_reservation_participants | ( | container_type & | results, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 373 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::get_sessions_by_type | ( | container_type & | results, |
const string & | type | ||
) | const [inline] |
Definition at line 342 of file GroupSessionDatabase.h.
bool GroupSessionDatabase::is_member | ( | const uid_type | member, |
const gsid_type | gsid | ||
) | const [inline] |
Definition at line 425 of file GroupSessionDatabase.h.
References gsid().
unsigned int GroupSessionDatabase::remove_expired_reservations | ( | const sec_type | expires | ) | [inline] |
Definition at line 381 of file GroupSessionDatabase.h.
References expires().
unsigned int GroupSessionDatabase::remove_expired_sessions | ( | const sec_type | expires | ) | [inline] |
Definition at line 350 of file GroupSessionDatabase.h.
References expires().
static unsigned int GroupSessionDatabase::remove_member_by_uid | ( | PreparedStatement & | query, |
const uid_type | member | ||
) | [inline, static] |
Definition at line 125 of file GroupSessionDatabase.h.
static unsigned int GroupSessionDatabase::remove_members_by_uid | ( | PreparedStatement & | query, |
const iterator_type & | begin, | ||
const iterator_type & | end | ||
) | [inline, static] |
Definition at line 133 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::remove_observer_by_uid | ( | const uid_type | observer | ) | [inline] |
Definition at line 265 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::remove_observers_by_uid | ( | const iterator_type & | begin, |
const iterator_type & | end | ||
) | [inline] |
Definition at line 270 of file GroupSessionDatabase.h.
unsigned int GroupSessionDatabase::remove_reservation | ( | const gsid_type | gsid, |
const uid_type | creator | ||
) | [inline] |
Definition at line 356 of file GroupSessionDatabase.h.
References gsid().
void DatabaseWrapper::rollback_transaction | ( | ) | [inline, inherited] |
Definition at line 53 of file DatabaseTransaction.h.
References DatabaseWrapper::_database.
Friends And Related Function Documentation
friend class DatabaseTransaction [friend, inherited] |
Definition at line 38 of file DatabaseTransaction.h.
Member Data Documentation
Database DatabaseWrapper::_database [protected, inherited] |
Definition at line 39 of file DatabaseTransaction.h.
Referenced by DatabaseWrapper::begin_transaction(), DatabaseWrapper::end_transaction(), and DatabaseWrapper::rollback_transaction().
Definition at line 70 of file GroupSessionDatabase.h.
Definition at line 72 of file GroupSessionDatabase.h.
Definition at line 71 of file GroupSessionDatabase.h.
Definition at line 73 of file GroupSessionDatabase.h.
Definition at line 74 of file GroupSessionDatabase.h.
The documentation for this class was generated from the following file: